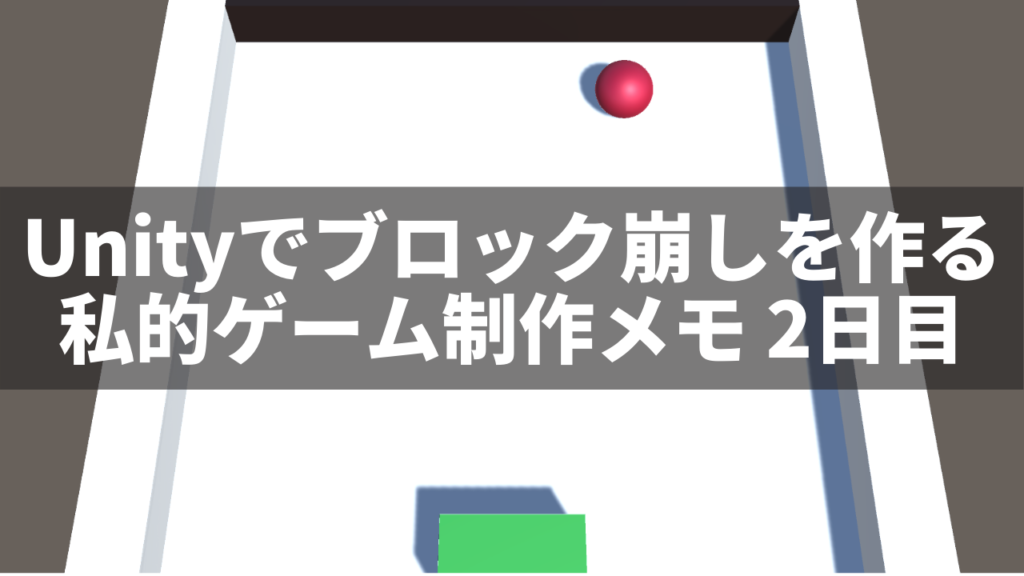
とりあえず何かゲームを作って見たほうが良いということだったので、下記動画を参考にUnityでブロック崩しを作ってみました。
結論から言うと作成できました。時間は3時間半ほどかかりました。
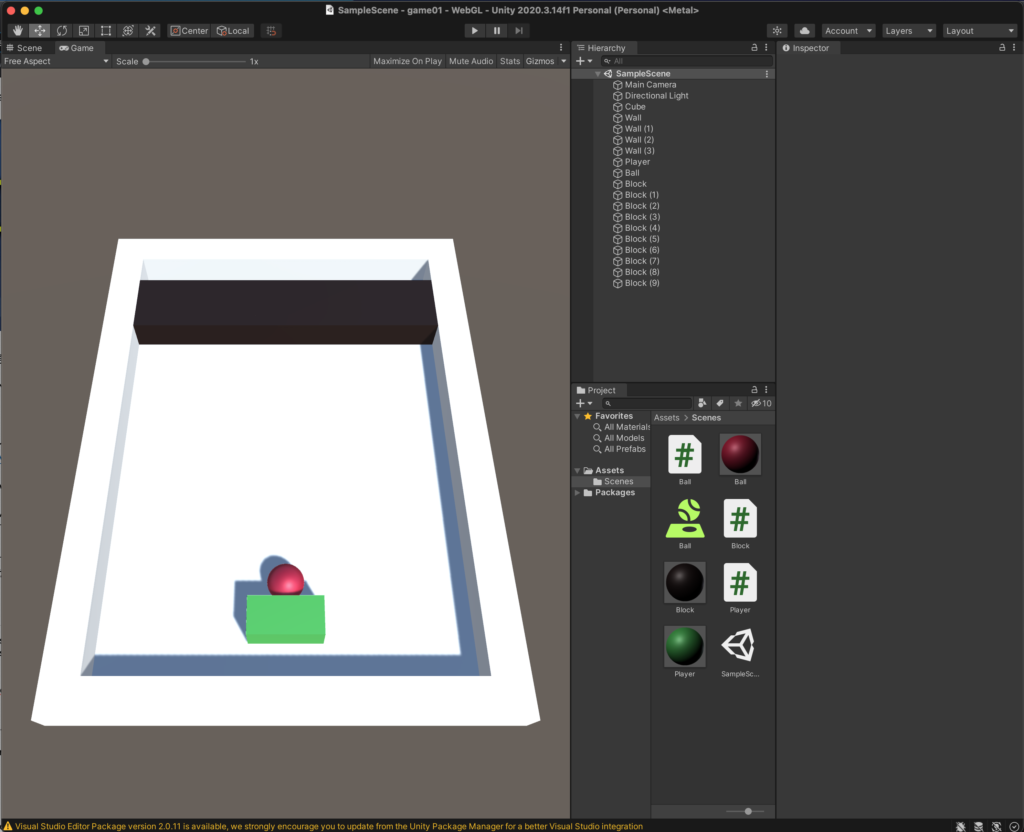
理由として、以下の2つのインストールに時間がかかったためです。
- Unityの最新版インストール
- Visual Studio for Macのインストール
作業的には2時間ぐらいで、一番時間がかかったのはコードの打ちミスの特定です。
上記動画では説明がないのですが、Visual Studioなどのコードエディタが無いとプログラムを打つのが大変です。ミスをしても気づかないですし、コードを一文字一文字チェックしていかないとうまく動きません。当たり前ですが。
自分が動画を見て書いたコードを載せておきます。
Player.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
public float speed = 1.0f;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
if (Input.GetKey(KeyCode.LeftArrow))
{
if (this.transform.position.x > -4)
this.transform.position += Vector3.left * speed * Time.deltaTime;
}
if (Input.GetKey(KeyCode.RightArrow))
{
if (this.transform.position.x < 4)
this.transform.position += Vector3.right * speed * Time.deltaTime;
}
}
}
Ball.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float speed = 1.0f;
private Rigidbody myRigid;
// Start is called before the first frame update
void Start()
{
myRigid = this.GetComponent<Rigidbody>();
myRigid.AddForce((transform.forward + transform.right) * speed, ForceMode.VelocityChange);
}
// Update is called once per frame
void Update()
{
}
}
Block.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Block : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
private void OnCollisionEnter(Collision collision)
{
Destroy(this.gameObject);
}
}